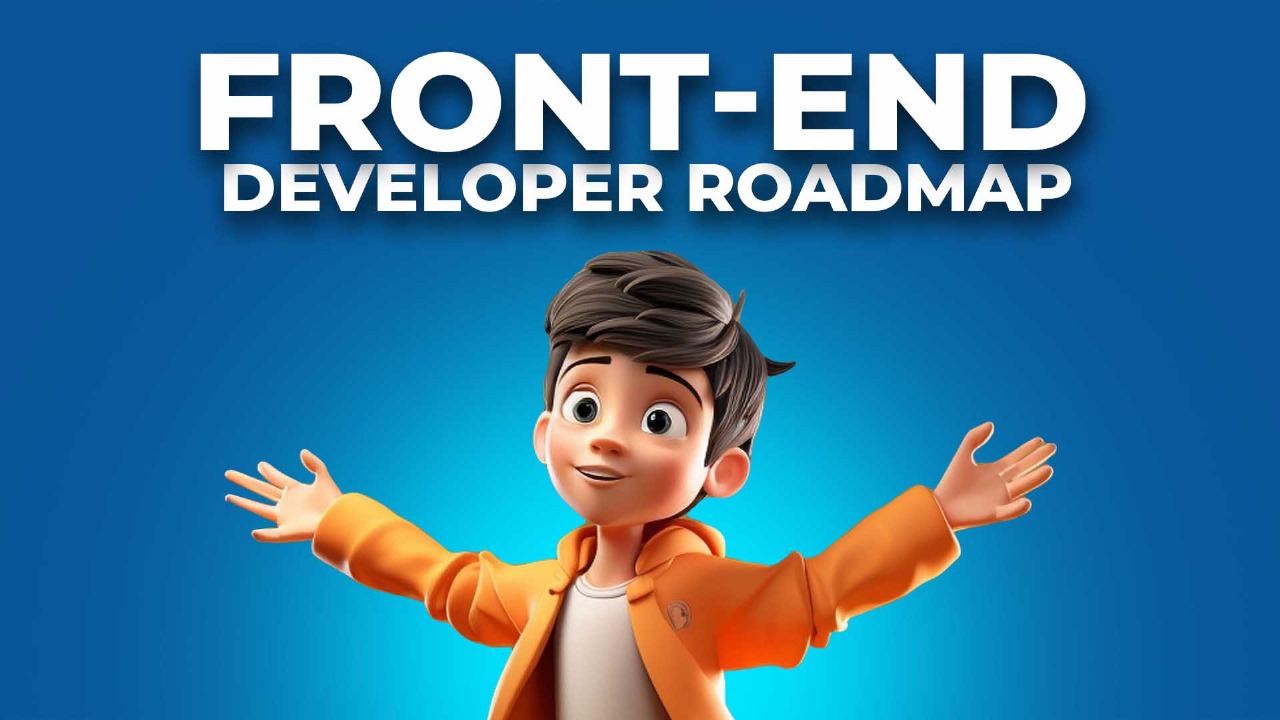
The role of a front-end developer is among the most sought-after in today’s tech-driven world. If you’re aspiring to become a front-end developer, understanding the roadmap can help you focus your learning and achieve your goals. This guide will provide a detailed, step-by-step overview of the skills, tools, and best practices required to master front-end development.
1. Understanding the Role of a Front-End Developer
What Does a Front-End Developer Do?
A front-end developer focuses on the user interface (UI) and user experience (UX) of a website or application. They are responsible for translating design files into functional, interactive web pages using code.
Key Responsibilities:
- Creating responsive layouts for various devices.
- Writing clean, efficient, and reusable code.
- Collaborating with designers and back-end developers.
- Optimizing website performance.
- Ensuring accessibility and cross-browser compatibility.
2. Basic Skills to Start With
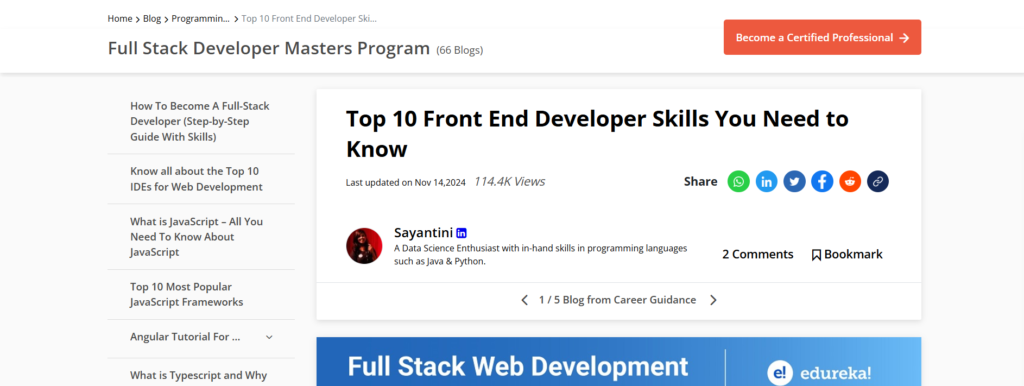
HTML (HyperText Markup Language)
HTML is the backbone of any web page. It structures the content, defining elements like headings, paragraphs, images, and links.
Key Concepts to Learn:
- Semantic HTML
- Forms and Inputs
- Accessibility (ARIA roles)
CSS (Cascading Style Sheets)
CSS is used to style HTML content. It controls the layout, colors, typography, and visual design of a website.
Key Concepts to Learn:
- CSS Box Model
- Flexbox and Grid Layout
- CSS Variables
- Media Queries for Responsive Design
JavaScript
JavaScript adds interactivity to web pages. It’s essential for creating dynamic content, handling events, and building complex UIs.
Key Concepts to Learn:
- DOM Manipulation
- Event Handling
- ES6+ Features (e.g., arrow functions, promises, modules)
- Fetch API and AJAX for Asynchronous Programming
3. Version Control and Collaboration
Git and GitHub
Version control is crucial for tracking changes and collaborating with others in a team environment.
Key Topics:
- Initializing a Git repository.
- Branching and Merging.
- Using GitHub for pull requests and code reviews.
4. Responsive Design and Mobile Optimization
Understanding Responsive Web Design
With the majority of web traffic coming from mobile devices, it’s essential to design for all screen sizes.
Tools and Techniques:
- Media Queries
- Fluid Grids
- Responsive Images (e.g., srcset)
Mobile-First Design
Adopt a mobile-first approach by designing and coding for smaller screens first and then scaling up for larger devices.
5. CSS Preprocessors
CSS preprocessors like Sass or Less can streamline your styling workflow by providing advanced features like nesting, mixins, and variables.
Why Learn CSS Preprocessors?
- They simplify complex CSS.
- Make your stylesheets more maintainable.
- Enable code reuse.
6. Build Tools and Automation
Task Runners
Automate repetitive tasks like minifying CSS and JavaScript, compiling Sass, or optimizing images with tools like:
- Gulp
- Grunt
Module Bundlers
Learn tools like Webpack, Parcel, or Vite to bundle JavaScript files and manage dependencies efficiently.
7. Frameworks and Libraries
CSS Frameworks
Frameworks like Bootstrap and Tailwind CSS speed up the development process by providing pre-designed components and utilities.
JavaScript Frameworks and Libraries
Familiarize yourself with popular JavaScript frameworks like:
- React (for building UI components).
- Vue.js (for progressive enhancement).
- Angular (for large-scale applications).
8. Testing and Debugging
Testing ensures the reliability of your code. Learn different types of testing:
- Unit Testing: Focus on individual components or functions using tools like Jest.
- Integration Testing: Test how different parts of the application work together.
- End-to-End Testing: Simulate real user interactions with tools like Cypress or Selenium.
Debugging is equally important. Use browser developer tools to identify and fix issues in your code.
9. API Integration
Learn how to consume APIs to fetch and display data dynamically. Skills to master include:
- RESTful APIs: Understanding HTTP methods (GET, POST, PUT, DELETE).
- JSON: Parsing and handling data.
- GraphQL: A modern alternative for querying APIs.
10. Accessibility (A11Y)
Creating accessible websites ensures inclusivity for users with disabilities.
Best Practices:
- Use semantic HTML.
- Add alt text for images.
- Ensure keyboard navigation.
- Test with screen readers.
11. Deployment and Hosting
Learn to deploy your projects to the web using platforms like:
- Netlify
- Vercel
- GitHub Pages
Understand hosting basics and familiarize yourself with CDNs (Content Delivery Networks) to improve website performance.
12. Soft Skills for Front-End Developers
Beyond technical expertise, soft skills play a significant role in your career growth.
Communication Skills
- Collaborate effectively with designers, back-end developers, and stakeholders.
- Convey technical concepts clearly.
Time Management
- Prioritize tasks and meet deadlines in a fast-paced environment.
Problem-Solving
- Break down complex issues and find efficient solutions.
13. Advanced Front-End Development Topics
Progressive Web Apps (PWAs)
PWAs provide a native app-like experience directly from the browser. They work offline and can be installed on devices.
Key Features to Learn:
- Service Workers
- Web App Manifest
- Caching Strategies
Web Performance Optimization
Optimizing web performance ensures fast loading times and better user experiences.
Techniques:
- Lazy Loading Images
- Code Splitting
- Minimizing HTTP Requests
- Using a Content Delivery Network (CDN)
Web Components
Web Components allow you to create reusable custom elements using:
- Shadow DOM
- HTML Templates
- Custom Elements API
14. Real-World Applications of Front-End Skills
Portfolio Websites
Showcase your work to potential employers or clients with an optimized and responsive portfolio.
E-commerce Sites
Learn to create user-friendly shopping experiences with interactive product pages and payment integrations.
Blogs and CMS Customization
Work on platforms like WordPress to create or customize themes for blogging purposes.
Single Page Applications (SPAs)
Build SPAs using frameworks like React or Angular to deliver faster, smoother user experiences.
15. Staying Up-to-Date with Front-End Trends
The front-end ecosystem evolves rapidly. Stay updated by:
- Following blogs like Smashing Magazine and CSS-Tricks.
- Joining communities on platforms like Reddit and Discord.
- Attending web development conferences and webinars.
16. Deep Dive into CSS Techniques
CSS is an essential skill for front-end developers, but advanced techniques and methodologies can take your skills to the next level.
Methodologies
CSS methodologies provide structured approaches to writing scalable and maintainable CSS.
Popular Approaches:
- BEM (Block Element Modifier): A naming convention for classes to make CSS more understandable and reusable.
- OOCSS (Object-Oriented CSS): Encourages code reusability and modularity.
- SMACSS (Scalable and Modular Architecture for CSS): Focuses on separating styles based on their purpose.
CSS Animations
Animations enhance the interactivity of your website.
Key Features to Learn:
- Using @keyframes for custom animations.
- CSS Transitions and Transformations.
- Animation libraries like Animate.css or GSAP.
Advanced Selectors
Master advanced CSS selectors to write efficient code:
- Attribute Selectors ([type=”text”])
- Pseudo-classes (:nth-child(), :hover, :not())
- Pseudo-elements (::before, ::after)
17. Advanced JavaScript Concepts
Functional Programming
Functional programming makes your JavaScript code more predictable and testable.
Key Concepts:
- Pure Functions
- Higher-Order Functions
- Immutability and Closures
Asynchronous JavaScript
Handling asynchronous operations is crucial in modern web development.
Tools and Techniques:
- Promises and Async/Await
- Working with APIs (REST and GraphQL)
- Error Handling and Debugging Asynchronous Code
JavaScript Design Patterns
Design patterns improve the structure and readability of your code.
Popular Patterns:
- Module Pattern
- Observer Pattern
- Singleton Pattern
18. Front-End Security Best Practices
Security is an integral aspect of web development to protect users and applications.
Common Vulnerabilities
- Cross-Site Scripting (XSS): Ensure user input is sanitized.
- Cross-Site Request Forgery (CSRF): Use anti-CSRF tokens.
- Clickjacking: Implement Content Security Policy (CSP).
Secure Coding Practices
- Avoid exposing sensitive data in client-side code.
- Use HTTPS to encrypt communications.
- Validate and sanitize user inputs on both client and server sides.
19. Building for Accessibility
WCAG Guidelines
The Web Content Accessibility Guidelines (WCAG) help developers create inclusive websites.
Core Principles:
- Perceivable: Information should be presented in a way all users can perceive.
- Operable: All functionalities must be accessible via keyboard and assistive technologies.
- Understandable: Content should be readable and predictable.
- Robust: Websites must be compatible with current and future user tools.
Testing for Accessibility
Use tools like Lighthouse, Axe, or WAVE to identify and resolve accessibility issues.
20. Monitoring and Maintenance
Performance Monitoring
Ensure your website remains fast and efficient post-deployment.
Tools:
- Google Lighthouse
- New Relic
- Pingdom
Error Logging
Use logging tools like Sentry to track and debug issues in real-time.
21. The Future of Front-End Development
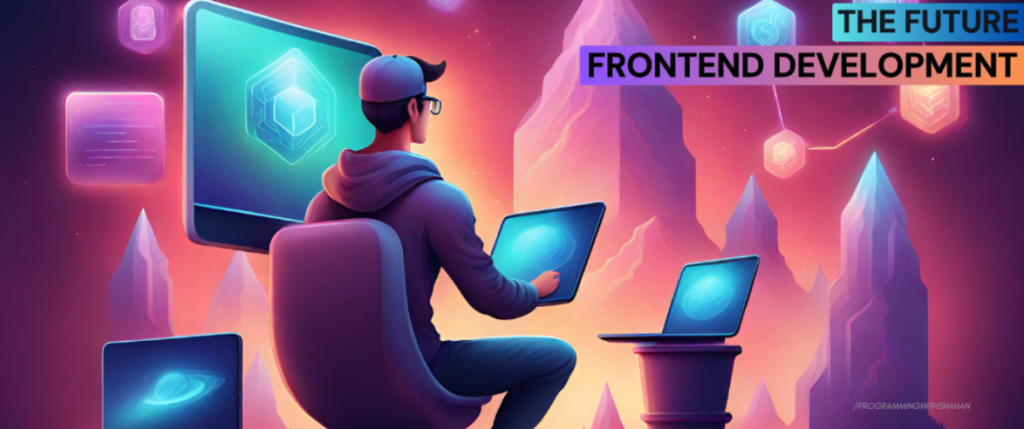
Web 3.0 and Blockchain Integration
With the rise of Web 3.0, front-end developers are increasingly working on decentralized applications (dApps) using blockchain technology.
AI-Powered User Interfaces
AI tools are enabling dynamic, predictive user interfaces that enhance user experience.
AR and VR in Web Development
Augmented Reality (AR) and Virtual Reality (VR) are shaping new dimensions in interactive web design.
22. Advanced Frameworks and Libraries for Front-End Developers
Modern front-end development is heavily reliant on frameworks and libraries that simplify complex tasks and enhance productivity. Let’s dive deeper into the most popular ones and their advanced use cases.
React.js
React is one of the most popular JavaScript libraries for building user interfaces, particularly SPAs (Single Page Applications).
Advanced React Concepts:
- Hooks: Leverage useState, useEffect, and custom hooks to manage state and side effects.
- Context API: Manage global state without prop drilling.
- Server-Side Rendering (SSR): Use frameworks like Next.js to improve performance and SEO.
- React Testing Library: Ensure your components function as expected with robust testing.
Vue.js
Vue is a progressive framework for building UIs, offering a simpler learning curve compared to React or Angular.
Advanced Vue Features:
- Vuex: Centralized state management for large applications.
- Composition API: A modern way of writing reusable logic.
- Nuxt.js: Framework for SSR and static site generation.
Angular
Angular is a complete framework ideal for building enterprise-level applications.
Advanced Angular Topics:
- RxJS: Master reactive programming for handling streams of data.
- NgRx: Implement state management in Angular apps.
- Lazy Loading Modules: Optimize performance by loading modules only when needed.
Svelte
Unlike other frameworks, Svelte shifts the work to compile time, resulting in faster runtime performance.
Key Features:
- Write less boilerplate code.
- Reactive declarations for automatic state updates.
- Minimal bundle sizes for optimized performance.
23. Static Site Generators and Jamstack
What is Jamstack?
Jamstack (JavaScript, APIs, Markup) is a modern architecture for building fast, scalable, and secure websites.
Benefits of Jamstack:
- Improved performance through static site generation.
- Enhanced security with no direct server dependencies.
- Scalable solutions using third-party APIs.
Popular Static Site Generators:
- Gatsby: Built on React and GraphQL for static site generation.
- Hugo: A fast and flexible static site generator using Go.
- Eleventy (11ty): Simple and powerful with support for multiple templating languages.
24. Front-End Tooling and Productivity Enhancements
Code Editors
Choose a robust code editor like Visual Studio Code and customize it with extensions for better productivity.
Essential VS Code Extensions:
- ESLint: Lint your JavaScript code.
- Prettier: Automatically format your code.
- Live Server: Preview your changes in real-time.
Browser Developer Tools
Mastering dev tools in browsers like Chrome and Firefox can streamline debugging and optimization.
Key Features:
- Inspect and edit HTML/CSS.
- Analyze network requests.
- Monitor JavaScript performance.
25. Becoming an Industry-Ready Front-End Developer
Building Real-World Projects
Apply your skills by working on meaningful projects:
- E-commerce website with payment gateway integration.
- Personal portfolio showcasing your expertise.
- Interactive dashboards using charting libraries like D3.js.
Contributing to Open Source
Collaborate on GitHub repositories to gain experience and visibility in the developer community.
Networking and Learning
Join front-end communities, attend webinars, and follow industry leaders to stay updated on trends.
26. Testing and Debugging in Front-End Development
Importance of Testing
Testing ensures your application is reliable, performs as expected, and delivers a seamless user experience.
Types of Testing
- Unit Testing: Tests individual components or functions.
Tools: Jest, Mocha, Jasmine. - Integration Testing: Verifies that different modules or services work together.
Tools: Cypress, Playwright. - End-to-End (E2E) Testing: Simulates real-world user scenarios to ensure the entire application works as intended.
Tools: Selenium, Puppeteer.
Debugging Techniques
Debugging helps identify and resolve errors in your code.
Key Tips:
- Use console.log effectively for initial debugging.
- Leverage browser dev tools to inspect errors and network activity.
- Write descriptive error messages for easier troubleshooting.
27. Deployment and Hosting for Front-End Applications
Popular Hosting Platforms
Choose a hosting platform that suits your project’s scale and complexity.
Options:
- Netlify: Ideal for static sites and Jamstack applications.
- Vercel: Best for Next.js and React applications.
- AWS Amplify: A robust solution for scalable applications.
- Firebase Hosting: Provides fast and secure hosting with integrated tools.
Continuous Integration and Deployment (CI/CD)
Automate the deployment process for quicker updates and seamless rollouts.
Popular CI/CD Tools:
- GitHub Actions
- CircleCI
- Travis CI
28. Career Growth and Opportunities in Front-End Development
Job Roles
Front-end development opens doors to various roles in the tech industry.
Examples:
- Front-End Developer: Focuses on building user-facing applications.
- UI/UX Developer: Works at the intersection of design and development.
- Web Animator: Specializes in creating interactive animations.
- Accessibility Specialist: Ensures websites are accessible to all users.
Freelancing and Entrepreneurship
Many front-end developers pursue freelancing or start their own agencies, offering services such as website creation and app development.
Certifications
Certifications can validate your skills and enhance your resume.
Popular Options:
- FreeCodeCamp Certifications.
- Google’s Mobile Web Specialist Certification.
- Microsoft Front-End Developer Certification.
29. Learning Soft Skills for Front-End Development
Communication Skills
Effective communication ensures seamless collaboration with designers, back-end developers, and clients.
Problem-Solving Abilities
Front-end development often involves debugging, optimization, and creative solutions to design challenges.
Time Management
Use productivity tools like Trello, Asana, or Notion to manage projects efficiently.
30. Final Words on the Front-End Developer Roadmap
Mastering front-end development is a journey that requires consistent learning and practice. From HTML basics to advanced frameworks and tools, each step builds a strong foundation for a successful career. Remember, the key is to stay curious, adapt to new technologies, and keep experimenting with innovative ideas.
Conclusion
Front-end development is the backbone of modern web applications, transforming complex ideas into user-friendly, visually appealing, and interactive interfaces. By following a well-structured roadmap, aspiring developers can navigate the vast landscape of tools, frameworks, and best practices.
The journey starts with mastering the fundamentals of HTML, CSS, and JavaScript before diving into advanced topics like frameworks (React, Angular, Vue), state management, and front-end tooling. Building accessible, secure, and performant websites is essential, as is staying updated with the latest trends like Jamstack, Progressive Web Apps (PWAs), and Web 3.0 technologies.
Success in this field demands not only technical proficiency but also soft skills like communication, problem-solving, and adaptability. With a growth mindset and hands-on experience through projects and collaboration, front-end developers can carve a rewarding career path in this ever-evolving domain.
Frequently Asked Questions (FAQs)
1. What is front-end development?
Front-end development involves building the user interface of websites and applications using technologies like HTML, CSS, and JavaScript. It focuses on creating a seamless and interactive user experience.
2. How long does it take to become a front-end developer?
The time it takes varies depending on your learning pace and dedication. For beginners, it typically takes 6 months to 1 year of consistent practice to gain a good grasp of the fundamentals and start building projects.
3. Do I need a degree to become a front-end developer?
No, a degree is not mandatory. Many front-end developers are self-taught or have completed online courses and bootcamps. What matters most are your skills, portfolio, and ability to solve real-world problems.
4. Which front-end framework should I learn first?
It depends on your goals, but React is a great starting point due to its popularity, community support, and extensive resources. Vue.js is beginner-friendly, while Angular is ideal for enterprise-level projects.
5. What tools are essential for front-end developers?
Some essential tools include:
- Code Editors: Visual Studio Code, Sublime Text.
- Version Control: Git and GitHub.
- Browser DevTools: Chrome, Firefox Developer Tools.
- Frameworks/Libraries: React, Vue, Angular.
- Design Tools: Figma, Adobe XD.
6. What are some common challenges in front-end development?
Common challenges include:
- Cross-browser compatibility issues.
- Debugging responsive designs.
- Optimizing performance.
- Managing state in large applications.
7. What is the difference between front-end and back-end development?
Front-end development focuses on the client-side (what users see and interact with), while back-end development handles the server-side (database management, application logic, and server configuration).
8. Is front-end development a good career choice?
Yes, front-end development is a promising career choice with high demand, competitive salaries, and opportunities to work in diverse industries.
9. How can I improve my front-end skills?
- Build real-world projects.
- Contribute to open-source repositories.
- Stay updated with industry trends.
- Participate in coding challenges.
- Follow tutorials and read documentation.
10. What is the future of front-end development?
The future includes advancements in Web 3.0, AI-driven interfaces, AR/VR integration, and the increasing adoption of Jamstack and serverless architectures. Staying updated and adaptable will be key.
This comprehensive roadmap is your guide to becoming a skilled front-end developer. Whether you’re starting from scratch or refining your existing skills, each section builds on the last, taking you closer to mastery.
Would you like me to continue expanding into specific technologies, such as React, advanced CSS techniques, or something else?
Explore more by joining me on IqBirds