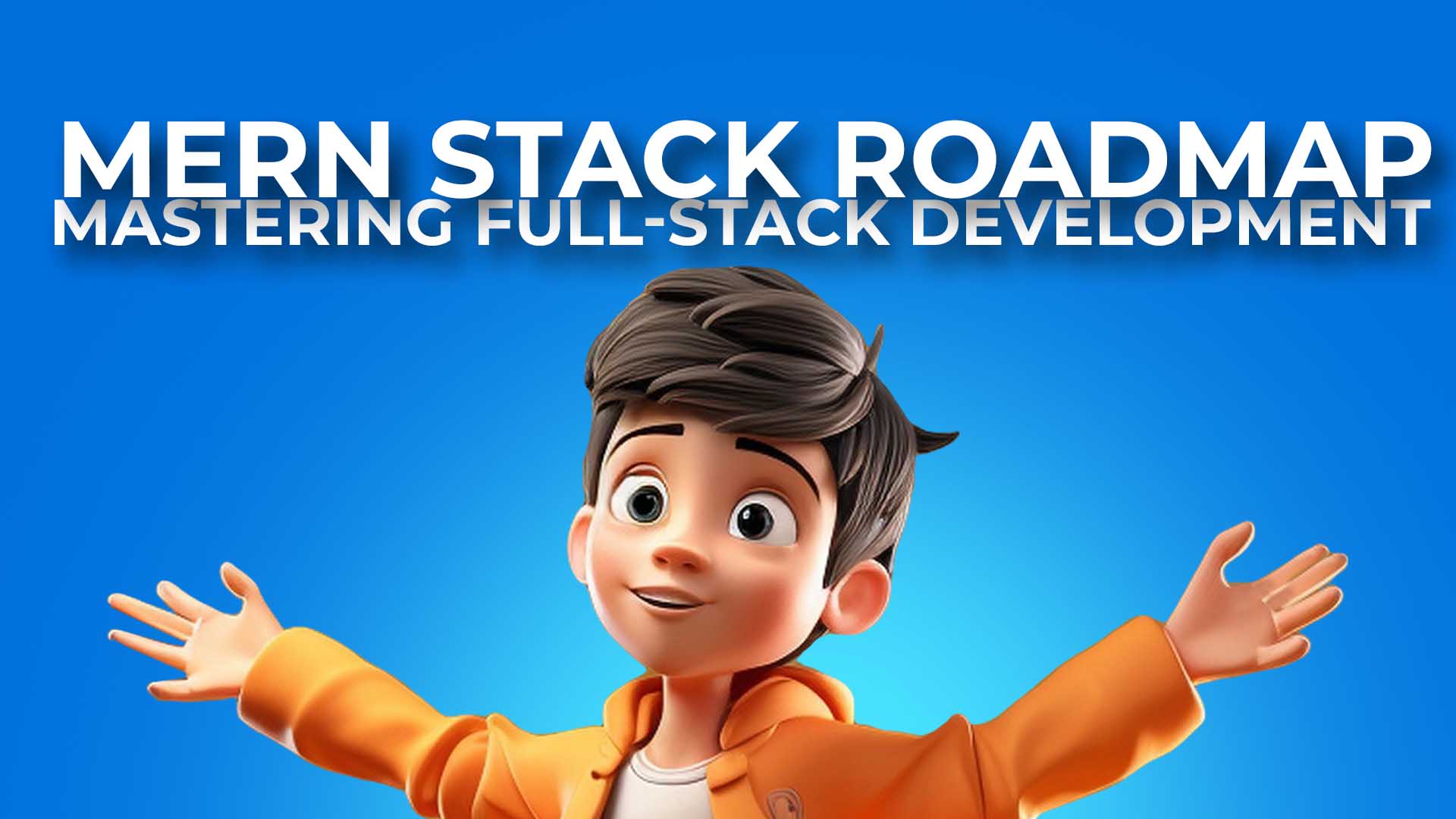
The MERN stack has become a popular choice for developers aiming to build robust, scalable, and efficient web applications. It combines four key technologies: MongoDB, Express.js, React.js, and Node.js, providing a seamless environment for both front-end and back-end development. In this article, we’ll outline a detailed roadmap to mastering the MERN stack, covering essential skills, tools, and best practices to help you excel in your development journey.
1. Introduction to the MERN Stack
The MERN stack is a powerful combination of technologies that allows developers to build full-stack applications using JavaScript from start to finish. By mastering this stack, you’ll be able to create dynamic web applications with a seamless user experience and efficient server-side logic.
2. Why Learn the MERN Stack?
Versatility and Efficiency
The MERN stack’s end-to-end JavaScript environment streamlines development and enhances productivity. Whether you’re creating single-page applications or complex systems, this stack offers flexibility and scalability.
High Demand in the Job Market
With the rise of web applications, MERN stack developers are in high demand. Mastering these technologies opens doors to lucrative career opportunities.
Community Support
Each component of the MERN stack is backed by a strong and active community. This ensures access to tutorials, forums, and resources that can guide you through challenges.
3. Understanding Each Component of MERN
MongoDB
MongoDB is a NoSQL database that stores data in a JSON-like format. It’s ideal for applications requiring a flexible schema and scalability.
- Key Features: Document-oriented, high scalability, and flexible query language.
- Use Cases: Real-time analytics, content management systems, and IoT applications.
Express.js
Express.js is a web application framework for Node.js that simplifies server-side development.
- Key Features: Middleware support, routing, and minimal setup.
- Use Cases: RESTful APIs, single-page applications, and back-end services.
React.js
React.js is a library for building dynamic user interfaces.
- Key Features: Component-based architecture, virtual DOM, and strong performance.
- Use Cases: Interactive web applications, dashboards, and e-commerce sites.
Node.js
Node.js allows JavaScript to run on the server, enabling full-stack JavaScript development.
- Key Features: Asynchronous programming, scalability, and npm ecosystem.
- Use Cases: Real-time applications, microservices, and API servers.
4. Prerequisites for Learning the MERN Stack
Before diving into the MERN stack, ensure you have a solid foundation in:
- HTML, CSS, and JavaScript: The building blocks of web development.
- Basic Programming Concepts: Variables, loops, functions, and data structures.
- Version Control Systems: Familiarity with Git and GitHub for code management.
5. Step-by-Step Roadmap
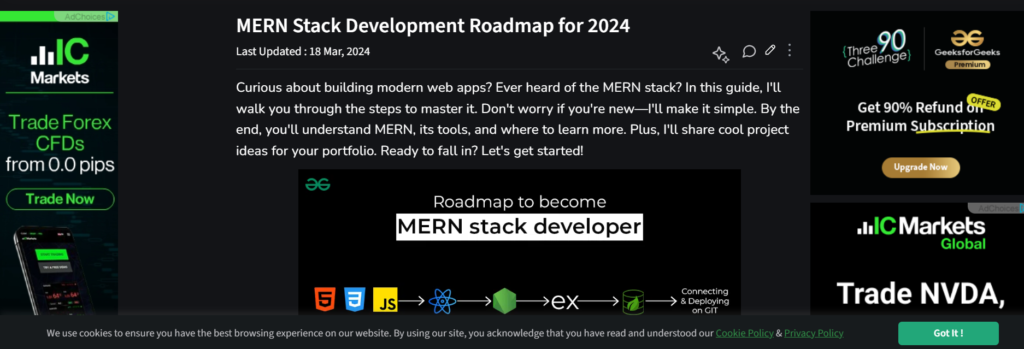
1: Basics of Web Development
- Learn the fundamentals of HTML, CSS, and JavaScript.
- Understand responsive design principles and CSS frameworks like Bootstrap or Tailwind CSS.
- Practice building static web pages.
2: Front-End Development with React
- Study React fundamentals: components, props, and state.
- Learn how to manage application state using Redux or Context API.
- Build small projects like a to-do list or weather app to apply your skills.
3: Back-End Development with Node.js and Express.js
- Start with Node.js basics: modules, event loop, and file handling.
- Dive into Express.js to understand routing, middleware, and RESTful APIs.
- Practice by creating APIs and connecting them with your front-end projects.
4: Database Management with MongoDB
- Learn how to set up and configure MongoDB.
- Understand CRUD operations and schema design.
- Practice by integrating MongoDB with Express.js to build a simple API.
6. Advanced Topics in the MERN Stack
Once you’re comfortable with the basics, explore these advanced topics:
- Authentication and Authorization: Use tools like JWT and OAuth for secure applications.
- Real-Time Communication: Integrate WebSocket or libraries like Socket.IO for live updates.
- Performance Optimization: Learn techniques to improve application speed and scalability.
7. Building a Complete MERN Application
Bring everything together by building a full-stack project. For instance:
- A blogging platform with user authentication.
- A task management app with real-time updates.
- An e-commerce site with a shopping cart and payment integration.
8. Deployment Strategies for MERN Applications
Once you’ve built your MERN stack application, the next step is to deploy it so that users can access it online. Deployment involves hosting both the front-end and back-end of your application, along with your database. Here’s how you can approach this:
1. Setting Up a Hosting Service
- Choose a hosting platform like Heroku, Vercel, Netlify, or AWS.
- For the back-end (Node.js and Express.js), platforms like Heroku or AWS work well.
- For the front-end (React.js), Vercel and Netlify are popular choices for their seamless integration with Git repositories.
2. Database Hosting
- Use MongoDB Atlas for hosting your MongoDB database in the cloud. It offers a free tier suitable for development purposes.
- Configure the database connection string in your application and ensure environment variables are securely managed.
3. Build and Deploy the Front-End
- Use npm run build to create a production-ready build of your React application.
- Upload the build folder to your hosting service or link your GitHub repository for automatic deployments.
4. Deploy the Back-End
- Ensure your Express.js server is production-ready by configuring proper CORS settings and securing API keys.
- Deploy the back-end code to a hosting service, and test API endpoints to ensure they are functioning correctly.
5. Configure a Proxy
- If you’re hosting the front-end and back-end on different platforms, set up a proxy in your React app or use tools like NGINX to manage traffic.
6. Monitor and Maintain
- Use tools like PM2 to manage your Node.js processes.
- Implement monitoring solutions like New Relic or Datadog to track performance and detect issues.
9. Best Practices for MERN Stack Development
To ensure your projects are efficient, maintainable, and scalable, follow these best practices:
1. Maintain a Clean Codebase
- Use a consistent coding style with tools like ESLint and Prettier.
- Follow the DRY (Don’t Repeat Yourself) principle to avoid redundant code.
2. Secure Your Application
- Protect sensitive information using environment variables.
- Implement authentication and authorization mechanisms, such as JWT or OAuth.
- Validate user inputs to prevent security vulnerabilities like SQL injection or cross-site scripting (XSS).
3. Optimize Performance
- Use caching tools like Redis for frequently accessed data.
- Optimize database queries by indexing fields in MongoDB.
- Leverage React’s lazy loading and memoization techniques to enhance front-end performance.
4. Adopt Version Control
- Use Git for tracking changes and managing branches.
- Commit code regularly and write meaningful commit messages.
5. Test Your Application
- Use testing frameworks like Jest, Mocha, or Chai for unit and integration tests.
- Perform end-to-end testing with tools like Cypress or Selenium.
6. Write Comprehensive Documentation
- Document APIs with tools like Swagger.
- Provide clear instructions for setting up and running the project.
10. Common Challenges and How to Overcome Them
Even experienced developers face challenges while working with the MERN stack. Here are some common hurdles and tips to overcome them:
1. Integration Issues
When integrating the front-end with the back-end, issues like mismatched data formats or API errors may arise.
Solution: Use tools like Postman or Insomnia to test API endpoints before connecting them to the React application.
2. State Management Complexity
Managing complex application states in React can be challenging as the app grows.
Solution: Use state management libraries like Redux or React Query to simplify state handling.
3. Database Performance Bottlenecks
Large datasets can slow down MongoDB queries.
Solution: Optimize queries with indexes and limit the amount of data retrieved using pagination.
4. Deployment Errors
Deployment can be tricky, especially when dealing with environment variables and configuration settings.
Solution: Test the application locally with production settings before deploying and use CI/CD pipelines for smooth deployments.
5. Debugging Code
Tracking down bugs in a full-stack application can be time-consuming.
Solution: Use debugging tools like Chrome DevTools for the front-end and Node.js built-in debugger or VS Code’s debugging features for the back-end.
11. Real-World MERN Stack Project Ideas
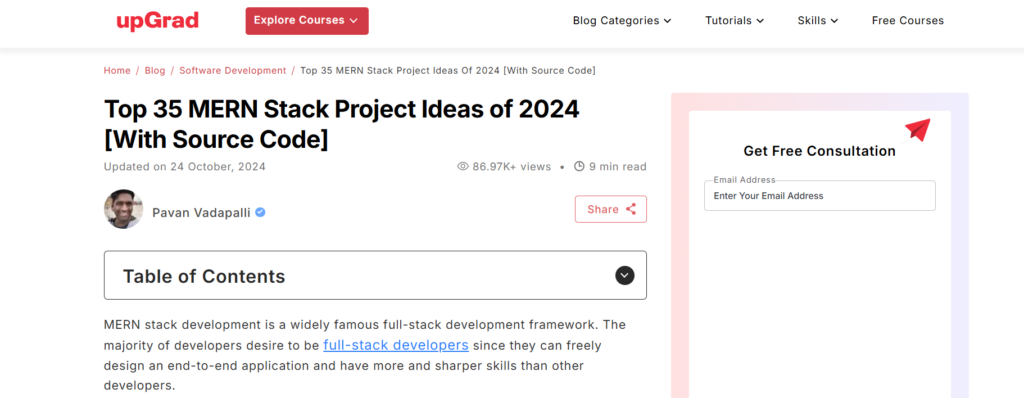
Building projects is one of the best ways to solidify your understanding of the MERN stack. Below are some real-world project ideas that cater to different levels of expertise, allowing you to apply the concepts you’ve learned.
Beginner Projects
- To-Do List Application
- Features: Add, update, and delete tasks with a simple UI.
- Purpose: Understand the basics of CRUD operations and React state management.
- Personal Blog
- Features: Create, edit, and delete posts with a backend API.
- Purpose: Work with MongoDB for data storage and React for rendering content dynamically.
- Contact Manager
- Features: Store and manage personal or professional contacts.
- Purpose: Practice form handling and basic authentication.
Intermediate Projects
- E-Commerce Platform
- Features: Product listing, cart functionality, and payment gateway integration.
- Purpose: Learn how to handle complex data structures and implement user authentication.
- Social Media Dashboard
- Features: User profiles, posts, likes, and comments.
- Purpose: Dive into relational data with MongoDB and build responsive designs with React.
- Event Management System
- Features: Create and manage events, RSVP options, and reminders.
- Purpose: Explore full-stack form handling and notification systems.
Advanced Projects
- Online Learning Platform
- Features: User authentication, video streaming, and progress tracking.
- Purpose: Implement advanced features like file uploads and secure APIs.
- Real-Time Chat Application
- Features: One-to-one and group chats, with typing indicators and read receipts.
- Purpose: Learn real-time data handling with Socket.IO and efficient database queries.
- Project Management Tool
- Features: Task assignment, progress tracking, and team collaboration.
- Purpose: Integrate advanced state management tools and role-based access control.
12. Advanced Topics in MERN Stack Development
Once you’ve built a few projects, delve deeper into advanced topics to refine your skills:
1. Microservices Architecture
- Break your application into smaller, manageable services.
- Use tools like Docker for containerization and Kubernetes for orchestration.
2. Serverless Functions
- Use services like AWS Lambda or Google Cloud Functions to run back-end logic without managing a server.
- Reduce costs and improve scalability for specific tasks.
3. Progressive Web Applications (PWAs)
- Transform your React application into a PWA for better offline support and faster load times.
- Use libraries like Workbox for service worker integration.
4. GraphQL Integration
- Replace REST APIs with GraphQL to fetch only the data your application needs.
- Tools like Apollo Client can simplify front-end integration.
5. DevOps for MERN Stack
- Learn CI/CD pipelines using Jenkins or GitHub Actions.
- Automate testing, building, and deployment processes.
13. Resources for Further Learning
Here are some resources to expand your knowledge and skills with the MERN stack:
Books
- Learning React: Functional Web Development with React and Redux by Alex Banks and Eve Porcello.
- Node.js Design Patterns by Mario Casciaro and Luciano Mammino.
Online Tutorials
- FreeCodeCamp’s MERN stack guide.
- The Odin Project’s full-stack curriculum.
Courses
- Udemy: The Complete React Developer in 2023 by Maximilian Schwarzmüller.
- Coursera: Full-Stack Web Development with React by Hong Kong University.
Communities
- Reddit: r/webdev and r/javascript.
- Stack Overflow for coding challenges and solutions.
14. The Role of Testing in MERN Stack Development
Testing is a crucial aspect of MERN stack development, ensuring that your application is functional, reliable, and bug-free. With proper testing strategies, you can catch issues early and maintain a seamless user experience.
1. Types of Testing
- Unit Testing: Tests individual components or functions in isolation.
- Integration Testing: Verifies how different modules or components work together.
- End-to-End (E2E) Testing: Simulates real-world user interactions to ensure the entire application functions as intended.
- Performance Testing: Measures the application’s speed, responsiveness, and scalability under load.
2. Testing Tools for MERN Stack
- Jest: A popular testing framework for JavaScript and Node.js applications.
- Mocha and Chai: Ideal for back-end testing with Express.js.
- Cypress: Excellent for end-to-end testing of React applications.
- Supertest: Useful for testing HTTP requests in Express.js.
- MongoDB Memory Server: Allows testing with an in-memory MongoDB instance, ensuring faster and isolated tests.
3. Best Practices for Testing
- Automate tests as much as possible to save time and reduce human error.
- Write clear and descriptive test cases for each functionality.
- Use mocks and stubs to isolate the unit under test.
- Regularly run tests during development and as part of your CI/CD pipeline.
15. SEO and Optimization for MERN Applications
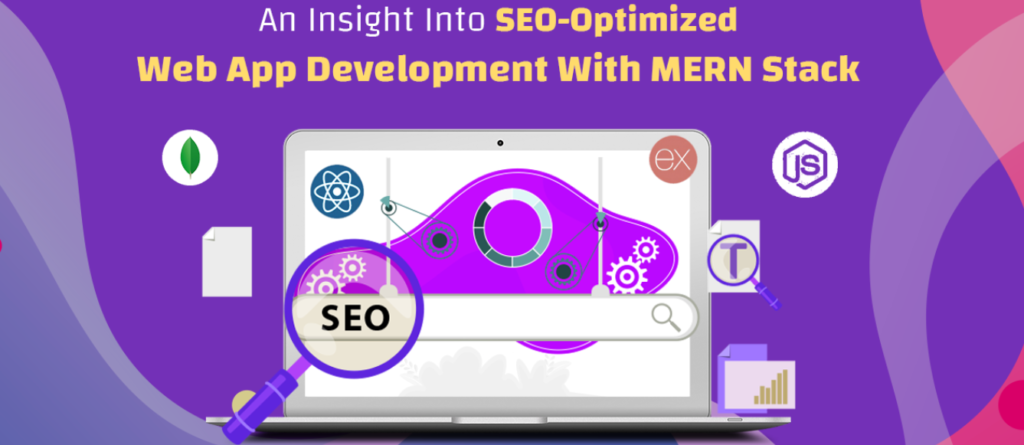
If your MERN stack application includes a front-end that’s accessible via search engines, Search Engine Optimization (SEO) becomes essential to improve visibility and attract users.
1. Server-Side Rendering (SSR)
React applications, by default, use Client-Side Rendering (CSR), which can hinder SEO since search engine crawlers struggle with JavaScript-heavy content. By implementing SSR using frameworks like Next.js, you can deliver HTML content pre-rendered on the server, boosting SEO.
2. Optimize Meta Tags and URLs
- Use tools like react-helmet to manage meta tags dynamically in React applications.
- Ensure URLs are clean, descriptive, and user-friendly.
3. Image Optimization
- Use responsive images and modern formats like WebP for faster load times.
- Implement lazy loading for images using libraries or native browser support.
4. Mobile-Friendly Design
- Ensure your application is fully responsive and works seamlessly on all devices.
- Use React’s flexbox and grid features for adaptive layouts.
5. Improve Performance
- Use code-splitting and lazy loading to reduce the initial load time.
- Minify JavaScript and CSS files to improve page speed.
16. MERN Stack in the Job Market
Learning the MERN stack opens doors to a variety of career opportunities, as it’s widely adopted across industries. Here’s what to expect when pursuing a career in MERN development:
1. Roles and Responsibilities
- Front-End Developer: Focus on building dynamic user interfaces with React.
- Back-End Developer: Work on creating APIs, managing servers, and database operations.
- Full-Stack Developer: Handle both front-end and back-end development, integrating all components seamlessly.
2. Skills Employers Look For
- Proficiency in JavaScript, React, Node.js, and MongoDB.
- Familiarity with version control systems like Git.
- Experience with deployment platforms and CI/CD pipelines.
- Knowledge of performance optimization and security best practices.
3. Tips for Landing a Job
- Build a strong portfolio showcasing diverse MERN stack projects.
- Contribute to open-source projects to gain visibility and experience.
- Practice solving coding challenges on platforms like LeetCode or HackerRank.
4. Salary Expectations
MERN stack developers enjoy competitive salaries, with averages varying based on location and experience:
- Entry-Level: $60,000 – $80,000 per year.
- Mid-Level: $80,000 – $110,000 per year.
- Senior-Level: $110,000+ per year.
17. The Future of the MERN Stack
The MERN stack has proven to be a powerful combination for modern web development, and its popularity continues to grow. Let’s explore some trends and advancements that are shaping the future of the MERN stack.
1. Integration with AI and Machine Learning
- MERN stack applications are increasingly incorporating AI and machine learning to provide smarter user experiences.
- Tools like TensorFlow.js and Brain.js allow developers to integrate machine learning models directly into React or Node.js applications.
2. Adoption of Real-Time Capabilities
- Real-time applications like live chat, collaborative tools, and dashboards are becoming more common.
- With technologies like WebSockets and libraries like Socket.IO, developers can easily implement real-time features in MERN applications.
3. Cloud-Native Development
- The shift towards cloud-native architectures is pushing MERN developers to leverage serverless computing and microservices.
- Platforms like AWS, Azure, and Google Cloud provide tools to deploy MERN applications with high scalability and resilience.
4. Improved Developer Tools
- The React ecosystem is continually evolving, with new libraries and tools making development faster and more efficient.
- For example, React DevTools, Redux Toolkit, and Next.js are enhancing debugging, state management, and server-side rendering.
5. Expanding Community and Ecosystem
- The growing MERN community contributes to an ever-expanding collection of open-source tools, libraries, and best practices.
- Active forums, GitHub repositories, and developer conferences keep the stack up-to-date with industry trends.
18. MERN Stack vs. Other Full-Stack Technologies
The MERN stack is just one of many full-stack technologies. Let’s compare it with some alternatives to understand its unique advantages:
1. MERN vs. MEAN
- Key Difference: MEAN uses Angular instead of React for front-end development.
- Advantages of MERN: React offers a more flexible and modern approach compared to Angular, which has a steeper learning curve.
2. MERN vs. LAMP
- Key Difference: LAMP uses PHP, Apache, and MySQL instead of JavaScript-based tools.
- Advantages of MERN: The unified use of JavaScript across the stack simplifies development and allows for more dynamic and interactive applications.
3. MERN vs. JAMstack
- Key Difference: JAMstack focuses on pre-rendering and serverless architectures.
- Advantages of MERN: While JAMstack is optimized for static sites, MERN excels in building dynamic, data-driven applications.
4. MERN vs. Django with React
- Key Difference: Django uses Python for back-end development.
- Advantages of MERN: JavaScript-based tools in MERN allow for better compatibility and faster development cycles.
19. Challenges and Limitations of the MERN Stack
While the MERN stack is versatile, it’s not without its challenges. Here are some limitations to consider:
1. Steep Learning Curve
- Mastering the entire stack requires knowledge of four different technologies, which can be overwhelming for beginners.
2. Performance Bottlenecks
- Large-scale applications with complex data requirements may face performance issues if not optimized properly.
3. Lack of Built-In Security
- Developers need to implement security measures manually, such as protecting APIs and securing databases.
4. High Initial Setup Time
- Setting up and configuring the MERN stack can be time-consuming, especially for newcomers.
5. Scalability Concerns
- While MongoDB and Node.js support scalability, improper implementation can lead to bottlenecks in high-traffic scenarios.
20. Essential Tools and Libraries for MERN Development
Using the right tools and libraries can significantly enhance productivity and streamline MERN stack development. Below is a curated list of must-have resources:
1. Development Tools
- Visual Studio Code: A lightweight and highly customizable code editor with rich support for JavaScript and Node.js.
- Postman: Ideal for testing and documenting RESTful APIs during back-end development.
- Robo 3T: A MongoDB management tool to visualize and query your database easily.
2. Front-End Libraries
- Redux: Helps manage the state of complex React applications efficiently.
- Material-UI: A popular React component library for creating beautiful user interfaces.
- React Router: Simplifies navigation and routing in single-page applications (SPAs).
3. Back-End Libraries
- Express Middleware: Middleware like cors, helmet, and morgan improve API security, logging, and functionality.
- Mongoose: A powerful ODM (Object Data Modeling) library for MongoDB.
- Passport.js: Enables secure authentication with strategies like JWT and OAuth.
4. Testing Libraries
- Chai and Mocha: A perfect combination for testing Node.js applications.
- Jest: Comprehensive testing framework for both front-end and back-end testing.
- Enzyme: Specifically designed for testing React components.
5. Deployment Tools
- Heroku: A simple platform-as-a-service for deploying MERN applications.
- Netlify: Great for hosting static React front-end apps.
- Docker: Helps containerize your MERN stack for portability and scalability.
6. Productivity Enhancers
- ESLint and Prettier: Maintain code consistency and improve readability.
- Nodemon: Automatically restarts your server after detecting changes in the code.
- Webpack: A module bundler to optimize and package JavaScript files for deployment.
21. Debugging and Troubleshooting MERN Applications
Even the best developers encounter bugs. Efficient debugging strategies and tools can save countless hours during development.
1. Debugging Tools
- Chrome DevTools: Essential for inspecting front-end issues, monitoring network activity, and debugging JavaScript code.
- Node.js Debugger: Built-in debugging capabilities for server-side applications.
- Redux DevTools: Helps trace actions and state changes in React-Redux applications.
2. Common Issues and Fixes
- Database Connectivity: Verify MongoDB connection strings and ensure the database server is running.
- Cross-Origin Resource Sharing (CORS): Configure the cors middleware in Express to resolve CORS errors.
- Deployment Issues: Double-check environment variables and ensure build files are correctly generated and uploaded.
- React Errors: Use error boundaries in React to catch and handle runtime errors gracefully.
3. Best Practices for Debugging
- Use meaningful console logs and stack traces to identify the root cause of issues.
- Break down complex problems into smaller, testable components.
- Leverage unit and integration tests to catch bugs early.
22. Expanding Beyond the MERN Stack
While the MERN stack is incredibly versatile, combining it with other technologies can unlock new possibilities.
1. Adding TypeScript
- Replacing JavaScript with TypeScript in React and Node.js improves code quality and reduces runtime errors.
- Tools like ts-node and @types make it easy to integrate TypeScript into MERN applications.
2. Incorporating AI and Analytics
- Use TensorFlow.js or Python-based APIs to integrate machine learning features.
- Add analytics tools like Google Analytics or Mixpanel to monitor user behavior.
3. Integrating Third-Party APIs
- Incorporate payment gateways like Stripe or PayPal for e-commerce applications.
- Use APIs like Google Maps or OpenWeather to add interactive features.
4. Exploring Other JavaScript Frameworks
- Experiment with Next.js for server-side rendering or Svelte for a simpler front-end framework.
23. Deployment Strategies for MERN Applications
Deploying a MERN stack application is the final step in the development process, allowing users to access your project in a live environment. Let’s explore various deployment strategies and best practices.
1. Hosting Platforms
- Heroku: A beginner-friendly platform-as-a-service (PaaS) for deploying full-stack applications.
- Supports Node.js for the back end and allows you to serve React front-end files via the same server.
- Vercel: Ideal for deploying React applications with features like serverless functions.
- AWS and Google Cloud: Provide advanced capabilities for deploying scalable and secure MERN apps.
2. Steps to Deploy
- Prepare the Application:
- Build your React app using npm run build.
- Optimize the Node.js server for a production environment.
- Set Up a Cloud Database:
- Use MongoDB Atlas for a secure, cloud-hosted MongoDB instance.
- Deploy the Back-End:
- Use platforms like Heroku or AWS to deploy your Express and Node.js server.
- Serve the Front-End:
- Integrate React’s static files with your back-end server or deploy them on a separate service like Netlify.
- Monitor and Maintain:
- Use tools like New Relic or Datadog to monitor server health and application performance.
24. Conclusion
The MERN stack is a powerful, flexible, and efficient framework for developing modern web applications. Its unified JavaScript ecosystem simplifies development, making it a favorite choice for developers and organizations worldwide. From small-scale projects to large enterprise applications, MERN offers scalability, reliability, and a robust developer community for support.
Whether you’re a beginner stepping into full-stack development or a seasoned developer exploring new frameworks, mastering the MERN stack opens doors to endless possibilities. Its synergy with modern tools and trends like cloud computing, AI integration, and real-time features ensures that it remains a future-proof choice for developers.
FAQs
1. What is the MERN stack best used for?
The MERN stack is ideal for building dynamic web applications, single-page apps (SPAs), e-commerce platforms, real-time dashboards, and social media platforms. Its flexibility and scalability make it suitable for projects of any size.
2. Can I use the MERN stack for mobile app development?
Yes, you can use the MERN stack for mobile development by integrating it with frameworks like React Native. React Native allows you to reuse React components, making it easier to develop cross-platform mobile apps.
3. Is the MERN stack suitable for beginners?
Absolutely! While it may have a learning curve, the MERN stack is beginner-friendly due to its unified use of JavaScript. Numerous tutorials and resources are available to help new developers.
4. How do I secure a MERN application?
To secure a MERN app:
- Use HTTPS to encrypt data in transit.
- Implement authentication with tools like Passport.js or JSON Web Tokens (JWT).
- Sanitize user input to prevent SQL injection and XSS attacks.
- Regularly update dependencies to fix vulnerabilities.
5. How do I scale a MERN stack application?
To scale a MERN app:
- Use load balancers to distribute traffic.
- Opt for a cloud-hosted database like MongoDB Atlas for scalability.
- Implement caching strategies with tools like Redis.
- Use containerization with Docker for easier deployment.
Explore more by joining me on IqBirds